Blog
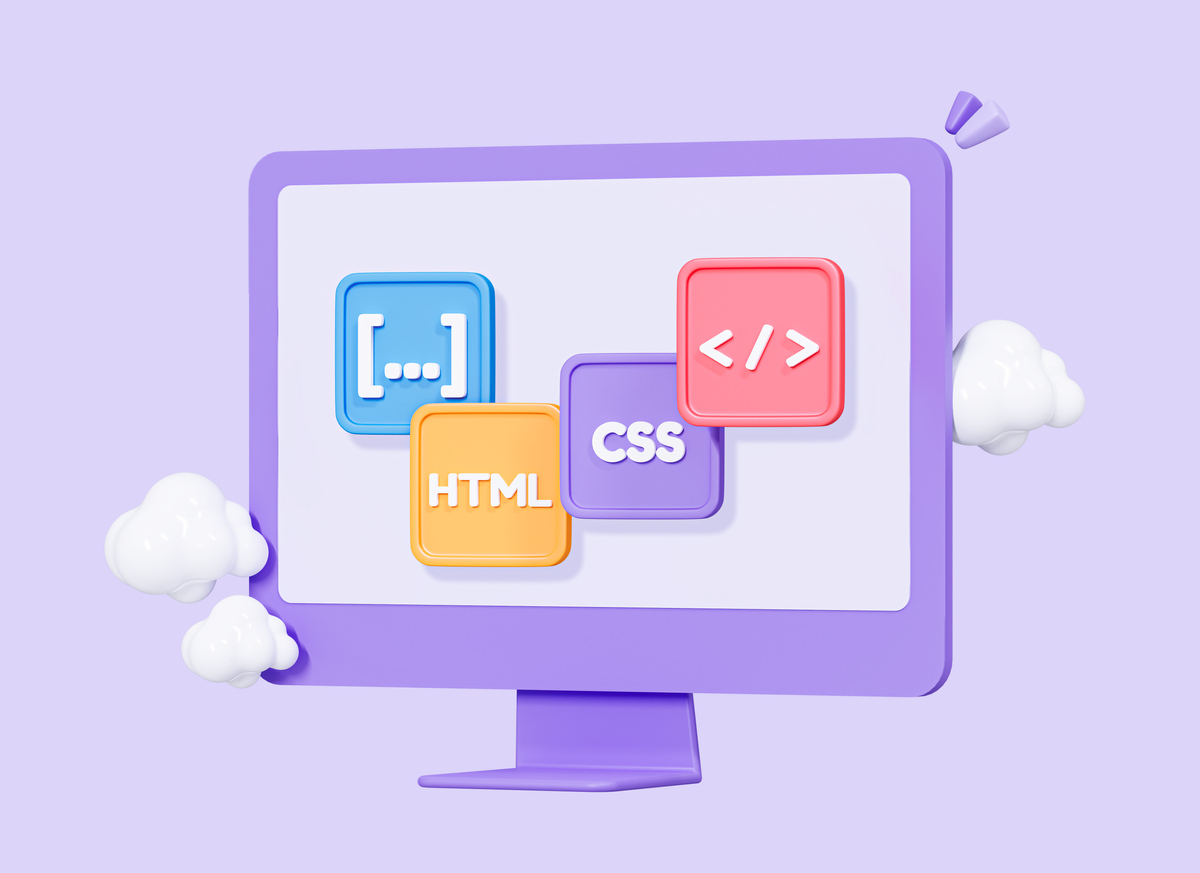
CSS best practises
Use a fallback font. By doing this you can ensure that even if a user's system doesn't have the preferred font, the browser will use the fallback font instead
body {
font-family: 'Comfortaa', sans-serif;
}
Use the DRY method: Don't repeat yourself. Try to evoid redundancies or repeating the same code. You can combine elements with same properties too
h1, h2, h3 {
font-family: 'Caveat', cursive;
color: blueviolet;
}
Avoid being too specific writing selectors, maybe there is no need to have extra selectors
/* Instead this: */
div ul li a {
color: pink;
text-decoration: none;
}
/* You can write this: */
li a {
color: pink;
text-decoration: none;
}
Use CSS Shorthand. This allows you to put multiple individual properties in a single line, making your CSS more concise and readable. You can use Shorthand with margins, paddings, borders, background, font, among others
/* Instead doing this */
h1 {
margin-top: 10px;
margin-right: 5px;
margin-bottom: 15px;
margin-left: 10px;
}
/* You can do this */
h1 {
margin: 10px 5px 15px 10px;
}
Keep it organized and consistent. Once you have your HTML, you can
write
your CSS from top to bottom of the website. Also, when you start naming your clases, try too be
consistent all over your files.
It's also a good practice put a line of separation between selectors
body {
/* Content here*/
}
h1 {
/* Content here*/
}
h2 {
/* Content here*/
}
main {
/* Content here*/
}
footer {
/* Content here*/
}
Use separate stylesheets for big projects. If you have different sections in your page with completely different styles you can create several stylesheets. This way you won't have to scroll for ever
<link rel="stylesheet" href="style/index.css">
<link rel="stylesheet" href="style/gallery.css">
<link rel="stylesheet" href="style/contact.css">
Use comments to make the file more readable and understandable. You can write comments to divide sections or explain certain decisions
/*Index.html css*/
/*About.html css*/
/*Media queries*/
It's important to know the differences between classes and IDs.
Classes are more versatile and can be applied to multiple elements, while
IDs are meant to unique and can only be used once per page.
IDs also have higher specificity, which can affect the order in which styles are applied when there
are
conflicting rules.
It's recommended to use classes for styling and reserve IDs for unique identifiers or JavaScript
interactions
/* CSS */
.class {
/* content here */
}
#id {
/*content here*/
}
<!--HTML-->
<!--Multiple elements with same class-->
<div class="container"></div>
<div class="container"></div>
<div class="container"></div>
<!--One single element with one unique ID, although it can have other classes too-->
<div id="contact-page" class="container"></div>