Blog
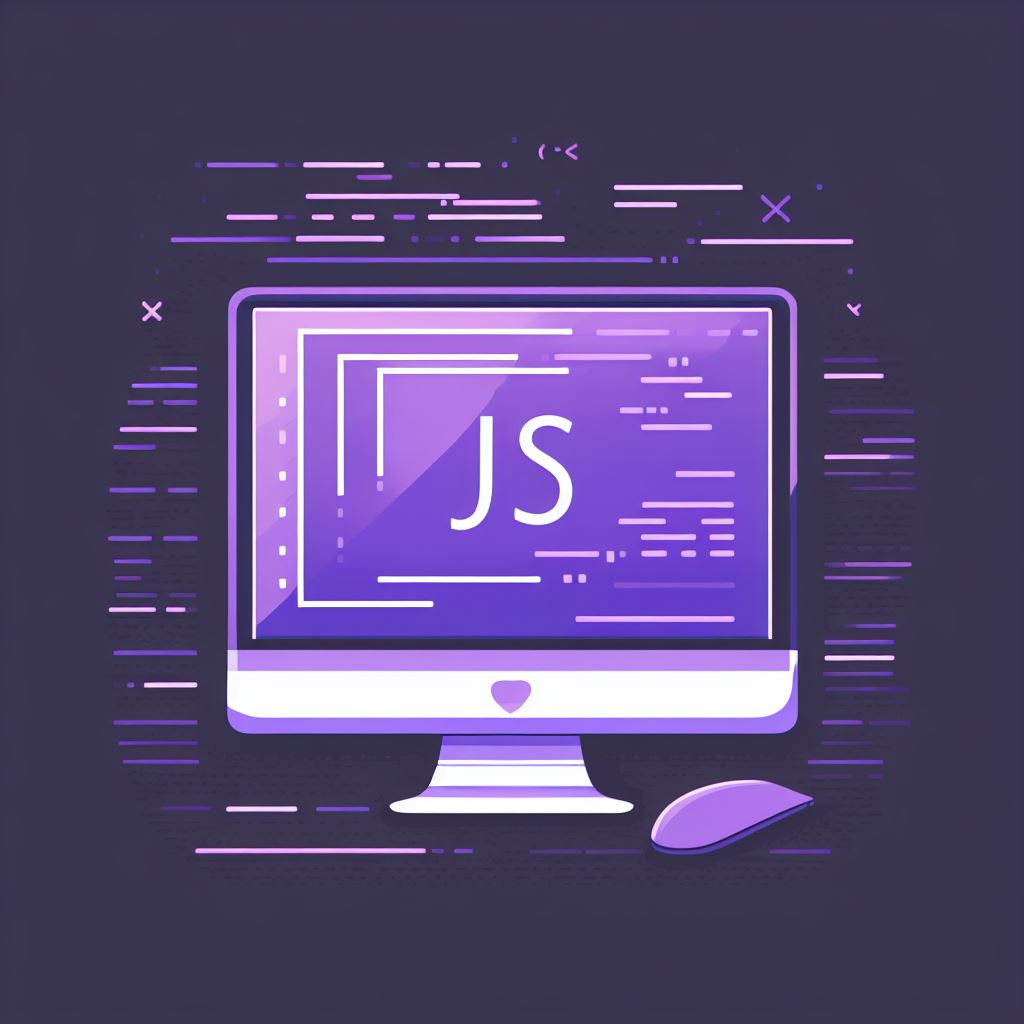
JS best practises
Use ===
Instead of ==
==
this is the abstract equality operator. If the operands are of
different
types,
JavaScript will try to convert one or both operands to a common type before making the comparison.
===
This is the strict equality operator, it always considers operands of
different types to be different.
Note: it's the same with the inequality operator
// abstract equality operator
2 == "2" // true
// strict equality operator
2 === "2" // false
// abstract inequality operator
2 != "2" // false
// strict inequality operator
2 !== "2" // true
Name variables and functions in a descriptive way that gives information about what the variable or function represents or stores, making the code more understandable, especially when working with a team
// non descriptive
let x = 25;
// descriptive
let numberOfStudents = 25;
Comment the code, which it will make the code more readbale and it will be easy to understand, providing additional context, explain the purpose of the code, or clarify something that might not be obvious
// Calculate the total price including tax
const calculateTotalPrice = (price, taxRate) => {
// Ensure the tax rate is in decimal form
const taxMultiplier = 1 + (taxRate / 100);
const totalPrice = price * taxMultiplier;
return totalPrice;
};
Put the JavaScript code in a different file and...
Code the script at the bottom of your HTML
<!-- ...content -->
</footer>
<script src="/src/index.js"></script>
</body>
</html>
Embracing let and const is a best practice in modern JS
- They have block scope, meaning they are only accessible within the block where they are defined
- They hey are not initialized until their declaration statement is encountered
- They can't be redeclared in the same scope. This helps prevent accidental redeclaration, reducing the risk of naming conflict
- Variables declared with const can't be reassigned, providing additional safety and clarity
// let
let x = 10;
x = 20; // Valid
// const
const y = 30;
// y = 40; // Error: Assignment to constant variable
// var (avoided in modern JavaScript)
var z = 50;
var z = 60; // No error, but generally considered poor practice
Code with error handling in order to anticipate, detect and prevent unexpected errors.
try {
// Code that may throw an error
const result = functionName();
} catch (error) {
// Handle the error
console.error(`Oops! An error occurred: ${error.message}`);
// Handle the error
}
Create unit tests following Test-Driven
Development (TDD). Unit test consists on test small pieces of code, like functions or methods.
TDD is a practice that consists on creating the unit tests before writing the actual code.
It helps to chatch bugs and issues, reduce errors and promotes writing modular and testable code
// Unit tests made with Jest
const { default: TestRunner} = require("jest-runner");
const bonusTime = require(`./bonusTime`);
test('if true, return the value plus 10, if false only the value', () => {
expect(bonusTime(1000, true)).toBe('€10000');
expect(bonusTime(200, false)).toBe('€200');
})
test('if salary is not a number, return a message', () => {
expect(bonusTime('1000', true)).toBe('Parameters must be a number and a boolean');
})
test('if bonus is not a boolean, return a message', () => {
expect(bonusTime(1000, 'true')).toBe('Parameters must be a number and a boolean');
})
test('if salary is negative, return a message', () => {
expect(bonusTime(-1, true)).toBe('Salary must be a positive number');
})
// Code
function BonusTime(salary, hasBonus) {
if (typeof(salary) !== "number" || typeof(hasBonus) !== "boolean" ) {
return 'Parameters must be a number and a boolean';
}
if (salary < 0) {
return 'Salary must be a positive number';
}
if (hasBonus === true) {
return `€${salary * 10}`;
} else {
return `€${salary}`;
}
}
module.exports = BonusTime;